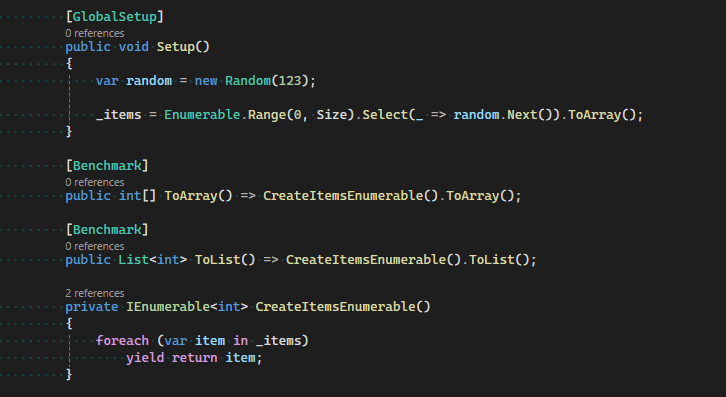
Last year I made an article comparing the performance of ToList versus ToArray when creating short lived collections that won’t be mutated, usually used to prevent multiple enumerations when iterating over a temporary LINQ transformation or to ensure mapping exceptions will be thrown inside the corresponding application layer.
The tests were performed with .NET Framework 4.8, .NET 7 and .NET 8, which concluded that ToArray
is significantly faster and more memory efficient for almost all collection sizes, with the only exception with very large collections in .NET 8 were ToList
was faster - but still uses more memory).
Assuming everything goes as planed, Microsoft should release .NET 9 by the end of 2024. This is the next major version of their most popular development framework that will bring a lot of new features (C# 13 is one of them) and performance improvements.