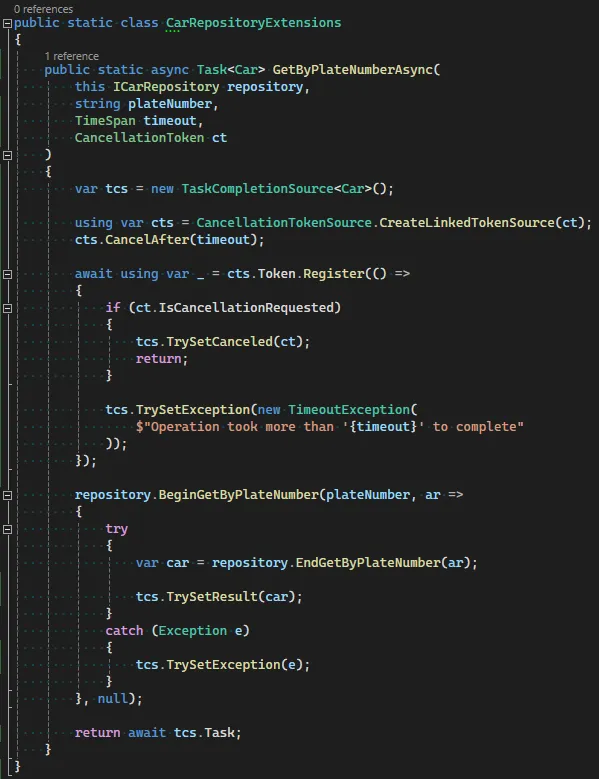
When Microsoft released .NET Framework 4.0 in April 2010, the Task Parallel Library (TPL) was introduced to help developers replace the previously used Asynchronous Programming Model (APM) pattern for a Task-based asynchronous programming.
Before the introduction of Tasks, when implementing asynchronous code, developers had to define two variations of the same method: one to begin the operation execution (convention: BeginOperationName
), that would receive an optional callback to be invoked when completed, and another to wait for the operation to complete (convention: EndOperationName
) and get the result or an exception, usually used inside the callback to prevent the main thread to be blocked.