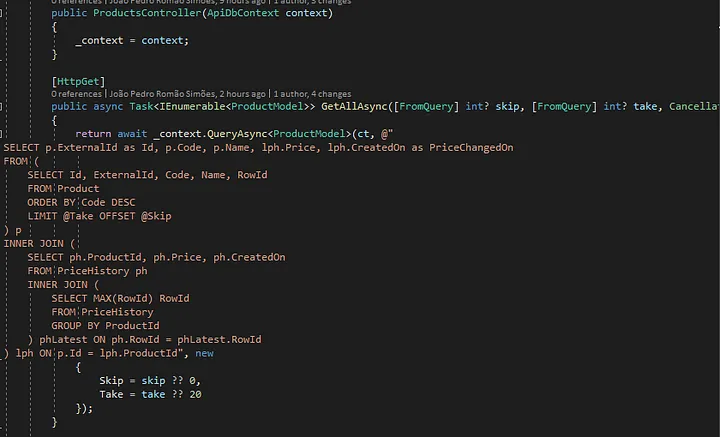
Nowadays it is extremely rare to implement an application without using any sort of library for Object-Relational Mapping (ORM) to reduce development time by removing the need to implement a lot of boilerplate code to access a database. In the .NET world that usually means using Entity Framework Core or NHibernate both offering strong tooling for CRUD operations, data type conversions, strong typed queries using LINQ with IQueryable
and so on.
Despite the pros of using an ORM there are also some cons that, while may not prevent them to be widely used inside an application, they may need to be replaced in some areas either for performance reasons or limitations. This usually means working directly with ADO.NET (oh hell no!) or use Micro ORM libraries, like Dapper, that are focused on performance and providing a simpler way to map database queries into objects.
In this article I’m going to demonstrate how Dapper can easily be integrated with Entity Framework Core (and probably with any other ORM) without using TransactionScope
, while trying to keep the same contracts via extension methods to DbContext
instances and ensuring the SQL is properly logged in a similar way to what EF Core usually does.